C Program To Count Repeated Character In A String
Continue reading C program to count frequency of each character in a string → Learn C programming, Data Structures tutorials, exercises, examples, programs, hacks, tips and tricks online. A blog for beginners to advance their skills in programming. C program to find the frequency of characters in a string: This program counts the frequency of characters in a string, i.e., which character is present how many times in the string. For example, in the string 'code' each of the characters 'c,' 'd,' 'e,' and 'o' has occurred one time.
- C Program To Count Number Of Same Characters In A String
- C Program To Count Repeated Character In A String Crossword Clue
I was trying to do a very common interview problem 'Finding the max repeated word in a string ' and could not find much resources in net for c/c++ implementation. So I coded it myself here. I have tried to do most of the coding from scratch for better understanding. Could you review my code and provide comments on my algorithm. Some people have suggested using hashtables for storing the count, but am not using hashtables here.
David Harkness3 Answers
$begingroup$Here's a long list of feedback. Sorry for the blunt tone, but I'm trying to be brief:
parsestr
mutates a global variable but returns a value that's relevant to that variable (the count). That's inconsistent. Either have it return both the count and the array (or make things easier on yourself and use avector
that knows its size), or make the count global too.You don't handle punctuation characters. Is that a requirement? Should
'a(b)' be one word? Should 'a. a' be two unique words?Do you need to split on characters other than space? What about
t
orn
? Other Unicode whitespace characters?Your
while(i < maxlength)
loop could more simply be afor
loop.Building up the word by incrementally contatenating characters onto a
string
is slow. It will periodically require dynamic allocation. A more efficient solution would be to remember the start index of the word. When you reach the end, create a string from the substring(start, end)
in one step.Going further from there, there's no reason to even store those wordsseparately since that start, end pair is enough to reconstruct it as needed.
Your program will mysteriously crash if you pass a sentence with more thanten words. :( Since you're using dynamic allocation everywhere else (
string
does that under the hood) there's no reason to use a fixed array for words.At the very least, you should have a constant for that size and check thatyou don't overflow the array.index
isn't a helpful variable name. Call itwordIndex
.Likewise,
wordcnt
would be better aswordCount
.maxrepeatedWord
is a mixture of camelCase and all lowercase. Be consistent(and camelCase is generally better since it makes it easier for the readerto split it into words).wordcntArr
would be better aswordCounts
. The fact that it's an array isself-evident.i <= count
should be just<
. Arrays are zero-based, sowordcntArr[count]
is past the last valid element.index
->indexOfMax
.You're passing
count
intocountwords
even though that count is relevant to a global variable that it accesses directly. Why not just make the count global too?The
else {}
accomplishes nothing. Remove it.If you declare
wordcnt
inside thefor(int i..
loop, you won't have toreinitialize it each iteration.int wordcntArr[10]
again duplicates the magical literal10
. Use a constant or, better, a dynamically-sized container.You're redundantly recounting each word every time it occurs. With
'I am am am good good'
, you'll get a count array like1 3 3 3 2 2
. If you had a collection of unique words instead, your $O(n^2)$ complexitywould go down to $O(mn)$ where $m$ is the number of unique words.A hash table would be a good way to create the set of unique words.
Your test string assumes repeated words will be contiguous (as opposed to,say
'I am good am good am.'
). Is that intentional? Handbook of textile auxiliaries and chemicals. Desirable?
At a high level, your algorithm is also not optimal. You've got $O(n^2)$ performance, ignoring the dynamic allocation as you incrementally build up those strings. With that, it gets worse.
I believe the canonical solution to this is (roughly):
That gives you $O(n)$: you only walk the entire string once.
esoteSome general comments first:
Comment, comment, comment. Although it seems pretty clear now, it doesn't hurt to make it really clear for the guy who has to maintain it when you've moved on.
You might want to have separate functions for doing the counting of the words and for displaying the counts of the words.
C++ Comments:
You are using C++, so you might want to put this in a Class.
The Standard Library is your friend. In particular, you might want to see if
std::map<..>
could make your life a little easier when counting words.The use of
string word[10]
makes an assumption of how many different words there will be. It would be better to allow an arbitrary number of different words by using something like astd::vector
. Alternatively, a different approach could be used (see #4).string.find(.)
andstring.sub_string(.)
might prove helpful.
There are two aspects of the problem statement that are unclear (that's quite probably intentional, given that this is an interview question).
What constitutes a 'word' here? Splitting the string on spaces is one possibility, but means that a word bounded by punctuation will be considered different to the same word standing alone. We can't completely ignore punctuation, though -
cant
andcan't
are completely different words, for example.What if there isn't a single most frequent word? There might be a draw, or there might be no words in the input at all. What should we return in those cases? (My recommendation: always return a container (e.g.
std::vector
) of results, and let the caller choose what to do if it's length is not 1.)
If you're not in a position to obtain answers to these questions, it's important to include comments indicating the assumptions you've made (i.e. your guesses at the answers).
Toby SpeightToby SpeightThis question already has an answer here:
- How would you count occurrences of a string (actually a char) within a string? 30 answers
I simply have a string that looks something like this:
'7,true,NA,false:67,false,NA,false:5,false,NA,false:5,false,NA,false'
All I want to do is to count how many times the string 'true' appears in that string. I'm feeling like the answer is something like String.CountAllTheTimesThisStringAppearsInThatString()
but for some reason I just can't figure it out. Help?
marked as duplicate by nawfal, PaRiMaL RaJ, luke, Fox32, jcwengerApr 25 '13 at 15:59
This question has been asked before and already has an answer. If those answers do not fully address your question, please ask a new question.
7 Answers
Chris BenardProbably not the most efficient, but think it's a neat way to do it.
rjdevereuxrjdevereuxC Program To Count Number Of Same Characters In A String
Your regular expression should be btrueb
to get around the 'miscontrue' issue Casper brings up. The full solution would look like this:
Make sure the System.Text.RegularExpressions namespace is included at the top of the file.
StuartLCThis will fail though if the string can contain strings like 'miscontrue'.
Sangram NandkhileC Program To Count Repeated Character In A String Crossword Clue
Here, I'll over-architect the answer using LINQ. Just shows that there's more than 'n' ways to cook an egg:
RobaticusRobaticusdo this , please note that you will have to define the regex for 'test'!!!
VoodooChild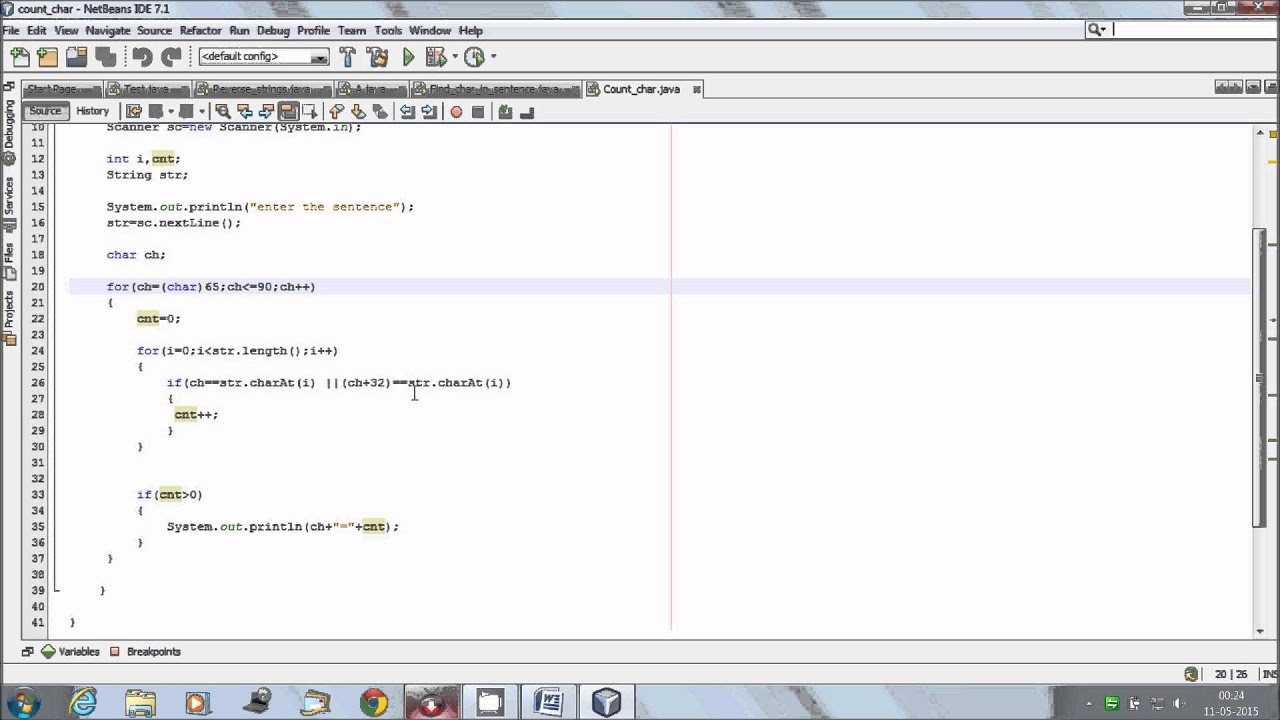